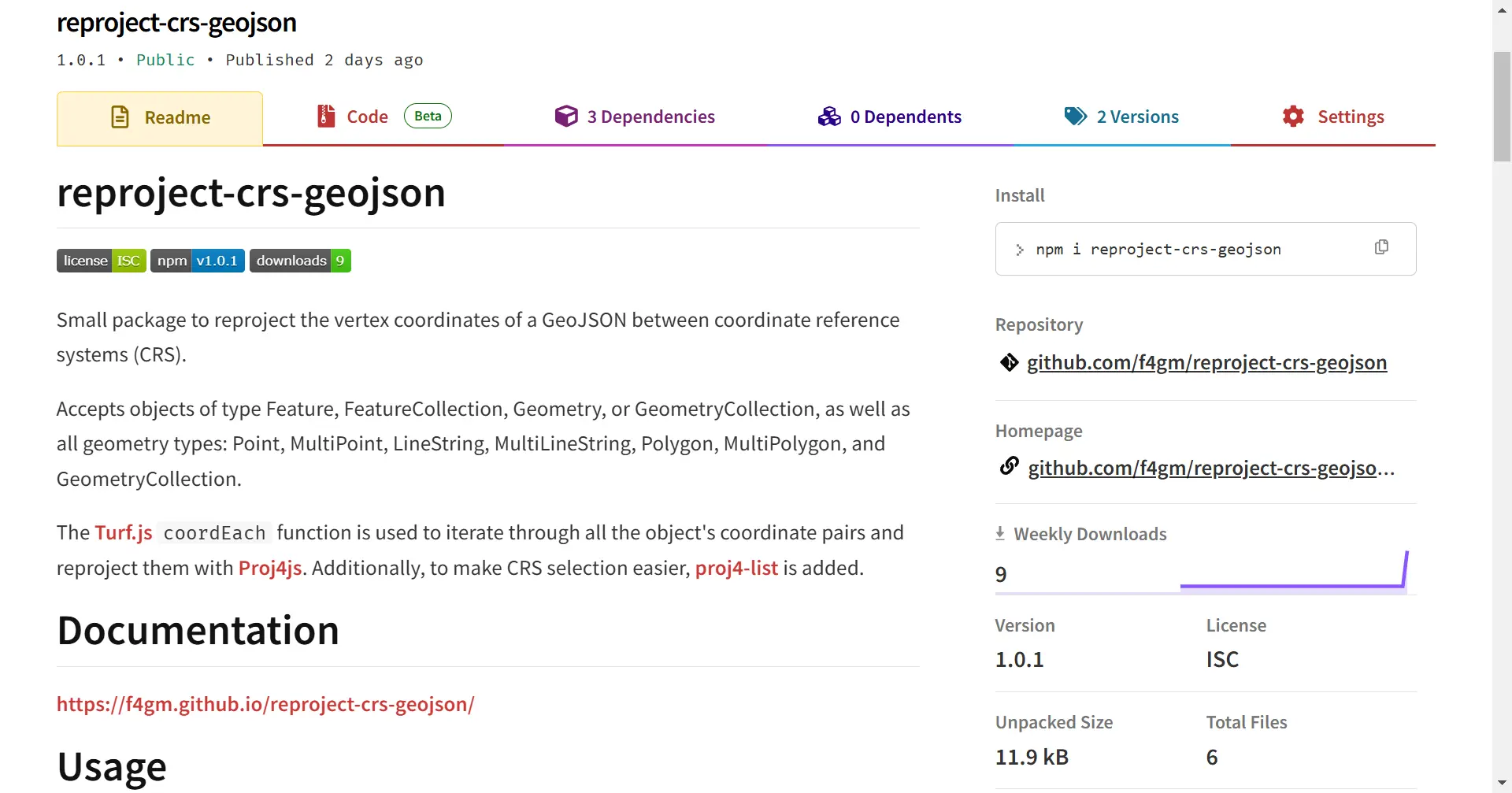
reproject-crs-geojson
reproject-crs-geojson es un pequeño paquete de NPM para reprojectar objetos GeoJSON entre sistemas de referencia de coordenadas (SRC).
- TypeScript
- Turf.js
- Proj4js
- proj4-list
reproject-crs-geojson
es una pequeña herramienta para reproyectar las coordenadas de los vértices de un GeoJSON entre distintos sistemas de referencia de coordenadas (SRC).
Admite objetos de tipo Feature, FeatureCollection, Geometry o GeometryCollection, así como todas las geometrías: Point, MultiPoint, LineString, MultiLineString, Polygon, MultiPolygon y GeometryCollection.
La función coordEach
de Turf.js se utiliza para recorrer todas las coordenadas del objeto y reproyectarlas con Proj4js. Además, para facilitar la selección del SRC, se incluye la biblioteca proj4-list.
Documentación
https://f4gm.github.io/reproject-crs-geojson/
Uso
Instalación del paquete
npm install reproject-crs-geojson
Importaciones
import {
reprojectGeoJSON,
getEPSG,
epsgExist,
toWGS84
} from "reproject-crs-geojson";
Reproyectar
La función reprojectGeoJSON
no afecta las propiedades, el tipo de geometría ni la cantidad de vértices; solo convierte el SRC.
import { reprojectGeoJSON } from "reproject-crs-geojson";
const Point = {
type: "Feature",
geometry: {
type: "Point",
coordinates: [1060479.7, 865010.7]
}
}
const converted = reprojectGeoJSON(
Point,
"EPSG:3115",
"EPSG:4326" // WGS84
);
console.log(converted);
// Expected
// {
// type: 'Feature',
// geometry: {
// type: 'Point',
// coordinates: [ -76.53327992507303, 3.3753051396728866 ]
// }
// }
También se puede incluir la cadena de texto PROJ.4:
import { reprojectGeoJSON } from "reproject-crs-geojson";
const epsg_3115 = "+proj=tmerc +lat_0=4.59620041666667 +lon_0=-77.0775079166667 +k=1 +x_0=1000000 +y_0=1000000 +ellps=GRS80 +towgs84=0,0,0,0,0,0,0 +units=m +no_defs +type=crs";
const Point = {
type: "Feature",
geometry: {
type: "Point",
coordinates: [1060479.7, 865010.7]
}
}
const converted = reprojectGeoJSON(
Point,
epsg_3115,
"EPSG:4326" // WGS84
);
Para convertir directamente a WGS84 (EPSG:4326), se puede usar la función toWGS84
:
import { toWGS84 } from "reproject-crs-geojson";
const epsg_3115 = "+proj=tmerc +lat_0=4.59620041666667 +lon_0=-77.0775079166667 +k=1 +x_0=1000000 +y_0=1000000 +ellps=GRS80 +towgs84=0,0,0,0,0,0,0 +units=m +no_defs +type=crs";
const Point = {
type: "Feature",
geometry: {
type: "Point",
coordinates: [1060479.7, 865010.7]
}
}
const converted = toWGS84(
Point,
epsg_3115
);
console.log(converted);
Utilidades EPSG
Para conocer si un código EPSG está en proj4-list:
import { epsgExist } from "reproject-crs-geojson";
console.log(epsgExist("foo"));
// false
console.log(epsgExist("EPSG:3115"));
// true
Si se requiere la cadena de texto PROJ.4:
import { getEPSG } from "reproject-crs-geojson";
console.log(getEPSG("EPSG:4326"));
// +proj=longlat +datum=WGS84 +no_defs
En teoría, según la especificación, los objetos GeoJSON deberían estar en el Sistema Geodésico Mundial 1984 (WGS 84). Sin embargo, en muchos casos, es necesario realizar este tipo de transformaciones.
Este es mi primer paquete de npm, así como mi primera incursión en el lenguaje TypeScript. Me disculpo de antemano por cualquier error.